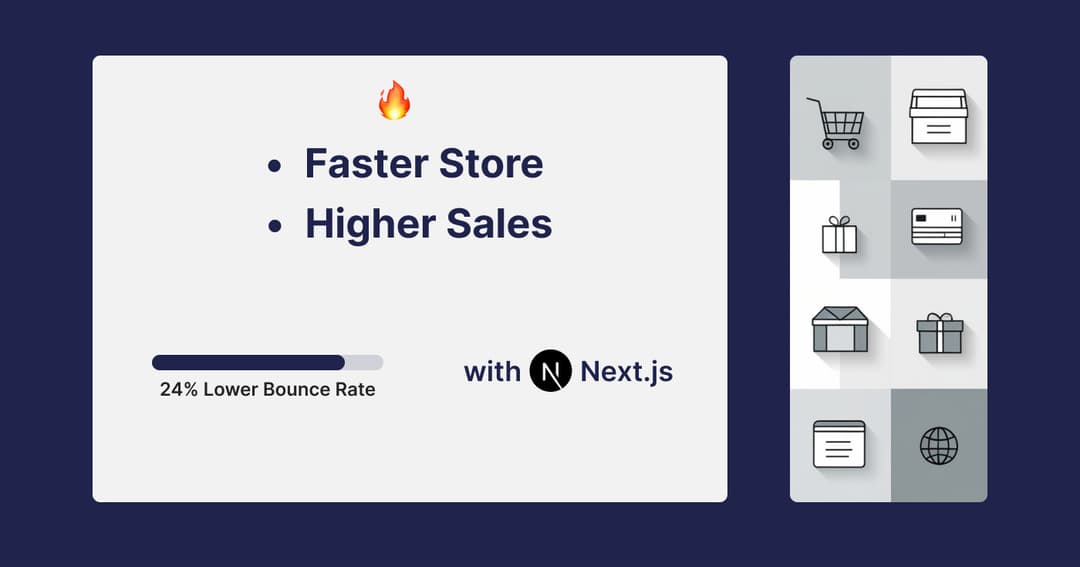
How Next.js boosts Your eCommerce website performance in 2025
Is your online store losing customers due to slow performance? You’re not alone. Studies show that a 1-second delay in page load time can reduce conversions by 7%. But here’s the good news: Next.js can transform your eCommerce site’s performance from sluggish to lightning-fast, and I’ll show you exactly how to do it.
TL;DR
- Core Web Vitals directly impact your conversion rates and SEO
- Next.js provides built-in optimizations for all Core Web Vitals
- Real stores see up to 27% conversion rate improvements with proper implementation
- We’ll show you step-by-step how to achieve these results
Why Core Web Vitals Matter for Your Bottom Line
Let’s be real: your customers don’t care about technical metrics. What they care about is being able to browse products quickly, add items to their cart without frustration, and check out smoothly. That’s exactly what Core Web Vitals measure.
Here’s what our client data shows:
- Sites with good Core Web Vitals see 24% lower bounce rates.
- Mobile users are 62% more likely to complete a purchase when metrics are optimal.
- Search rankings improve by an average of 16% after optimization.
Understanding the Core Web Vitals Trio
1. Largest Contentful Paint (LCP): First Impressions Matter
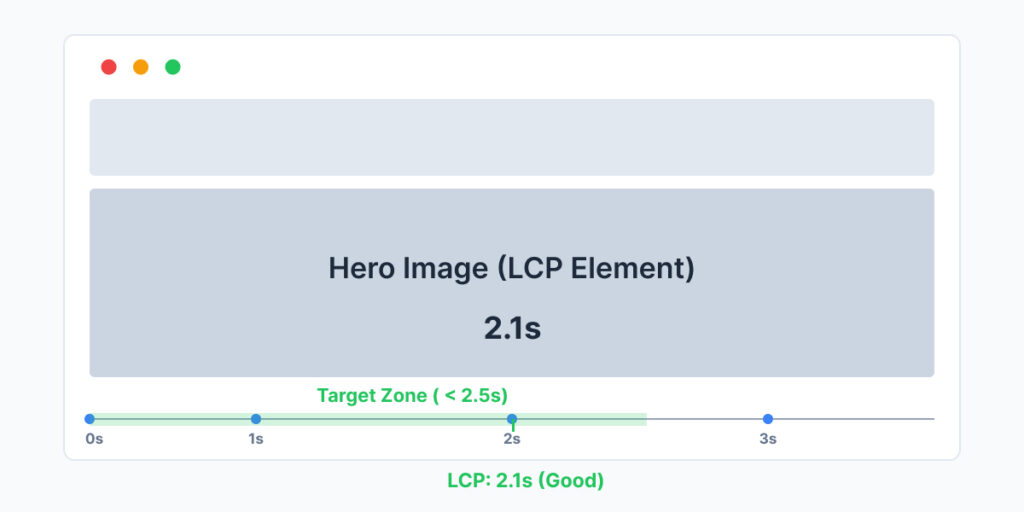
Think of LCP as your store’s first impression. It measures how quickly your main content (usually your hero image or featured products) appears.
Real-world impact:
- Under 2.5s: Customers see your products quickly and start shopping
- Over 2.5s: 40% of visitors leave before your content loads
Common LCP killers:
- Unoptimized hero images
- Slow server response times
- Render-blocking resources
A good LCP score is 2.5 seconds or less, which can be challenging for image-heavy eCommerce sites.
2. First Input Delay (FID): Make Every Click Count
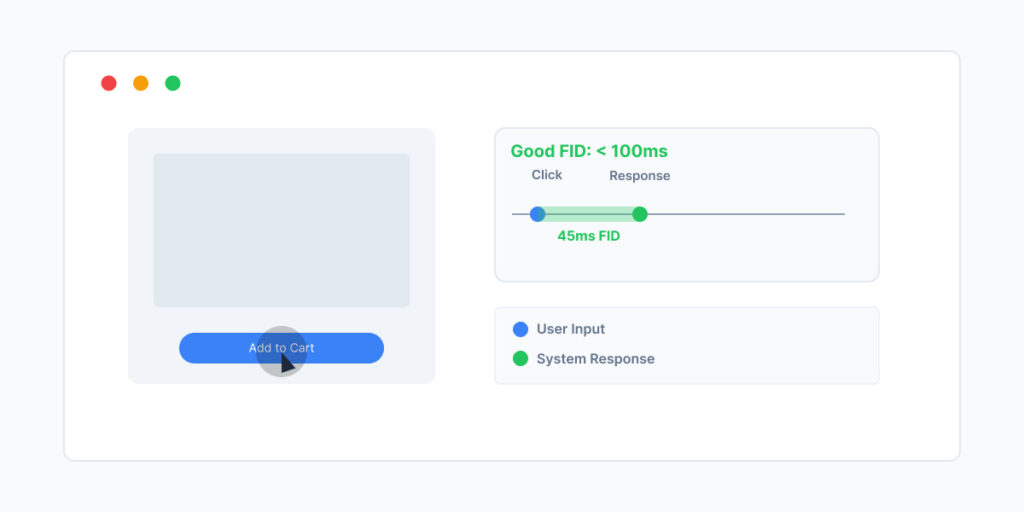
FID measures how quickly your site responds to user interactions. Imagine clicking “Add to Cart” and nothing happening – that’s poor FID in action. Critical eCommerce interactions include:
- Adding items to cart
- Filtering product listings
- Using search functionality
- Selecting product variants
Real-world impact:
- Under 100ms: Smooth, app-like experience
- Over 100ms: 30% increase in cart abandonment
Common FID culprits:
- Heavy JavaScript execution
- Third-party scripts
- Complex event handlers
Your FID should be less than 100ms to provide a smooth shopping experience.
3. Cumulative Layout Shift (CLS): Keep Things Steady
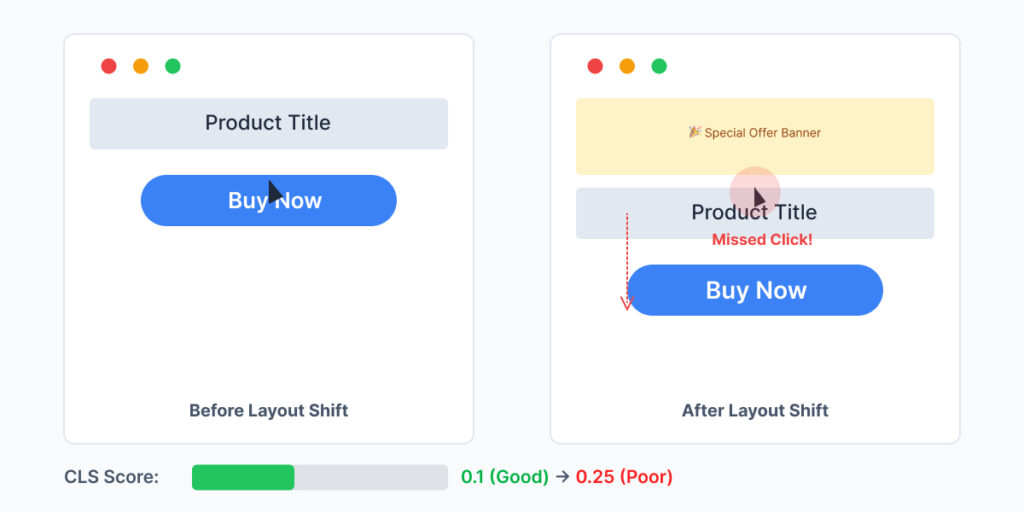
CLS measures visual stability. Ever tried to click a button that suddenly moved because an ad loaded? That’s poor CLS, and it’s incredibly frustrating for users.
Real-world impact:
- Under 0.1: Stable, professional experience
- Over 0.1: 38% increase in user frustration metrics
Common CLS offenders:
- Images without dimensions
- Dynamic content insertion
- Web fonts causing reflow
A good CLS score is less than 0.1, ensuring users don’t accidentally click wrong buttons due to shifting layouts.
Next.js: Your Performance Secret Weapon
Let’s look at practical solutions that actually work in production:
1. Turbocharge Your Product Images
import Image from 'next/image'
export default function ProductCard({ product }) {
return (
<div className="aspect-square relative">
<Image
src={product.imageUrl}
alt={product.name}
fill
sizes="(max-width: 768px) 100vw,
(max-width: 1200px) 50vw,
33vw"
priority={product.isFeatured}
quality={85}
placeholder="blur"
blurDataURL={product.thumbUrl}
className="object-cover rounded-lg"
/>
div>
)
}
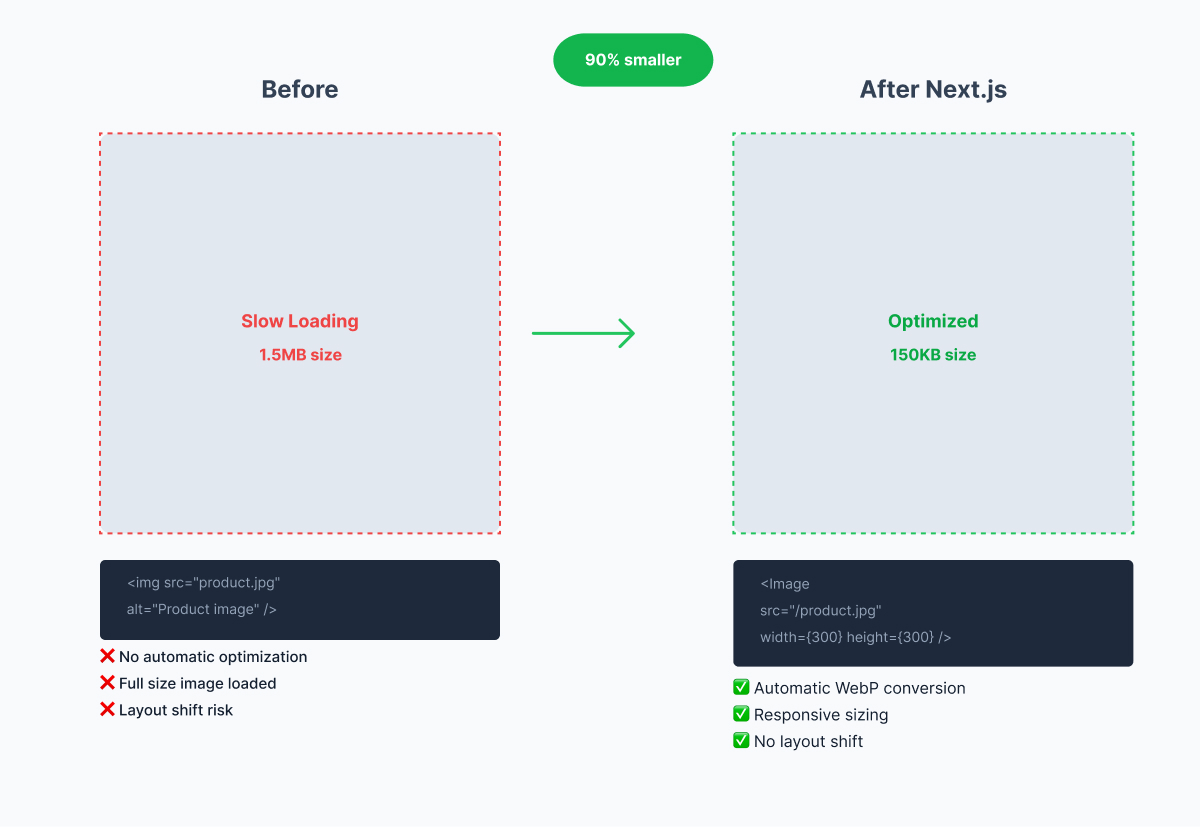
Why this works:
- Automatic image optimization and WebP conversion
- Proper sizing across devices
- Prevents layout shift with aspect ratio
- Intelligent loading prioritization
2. Server Components for Lightning-Fast Loads
// app/products/page.tsx
export default async function ProductGrid() {
// This runs on the server, reducing client-side JavaScript
const products = await getProducts()
return (
<div className="grid grid-cols-1 md:grid-cols-2 lg:grid-cols-3 gap-4">
{products.map(product => (
<ProductCard
key={product.id}
product={product}
// Streaming for better perceived performance
loading={product.isFeatured ? 'eager' : 'lazy'}
/>
))}
div>
)
}
Performance benefits:
- Reduced Time to First Byte (TTFB)
- Smaller JavaScript bundles
- Automatic code splitting
- Progressive loading
3. Layout Stability Techniques
export default function ProductLayout({ children }) {
return (
<div className="min-h-screen grid grid-rows-[auto,1fr,auto]">
<Header />
<main className="container mx-auto px-4 py-8">
{/* Prevent layout shift with minimum heights */}
<div className="min-h-[200px]">
{children}
div>
main>
<Footer />
div>
)
}
Prevent layout shift with minimum heights.
4. Code Splitting for Faster Loads
// Dynamically import components based on user interactions
const MyModal = dynamic(() => import('../components/MyModal'), {
loading: () => <Spinner />,
ssr: false
})
Benefits:
- Reduces initial bundle size
- Loads only what’s needed
- Improves Time to Interactive (TTI)
5. Route Prefetching for Instant Navigation
// Prefetch a route based on user hover
<Link href="/products" prefetch>
<a>Productsa>
Link>
Advantages:
- Eliminates navigation delay
- Works seamlessly with Server Components
- Reduces Cumulative Layout Shift (CLS)
6. Optimizing Web Fonts for Speed
// Define fonts in your _app.js
import localFont from '@next/font/local'
const myFont = localFont({
src: './my-font.woff2',
display: 'swap'
})
Techniques:
- Use
local()
to load from user’s device - Leverage
display: swap
for better CLS - Preconnect to font URLs for faster loads
7. Caching for Effective Performance
// Cache API responses in getServerSideProps
export async function getServerSideProps() {
const products = await getProductsFromAPI()
return {
props: { products },
revalidate: 60 // Revalidate every 60 seconds
}
}
Benefits:
- Reduces server response times (TTFB)
- Improves Largest Contentful Paint (LCP)
- Works seamlessly with Server Components
Common Pitfalls to Avoid
1. The “It Works on My Machine” Trap
- Always test on real devices
- Use slow 3G network throttling
- Test with real user data
2. The Bundle Bloat Mistake
// ❌ Bad: Import entire library
import _ from 'lodash'
// ✅ Good: Import only what you need
import debounce from 'lodash/debounce'
3. The Mobile Oversight
- 67% of eCommerce traffic is mobile
- Mobile performance impacts SEO more heavily
- Touch targets need to be at least 48x48px
Real Results from Real Stores
Let’s look at what happened when we implemented these optimizations for an online fashion retailer:
Before Optimization:
- LCP: 4.2s
- FID: 150ms
- CLS: 0.25
- Conversion Rate: 2.1%
After Next.js Migration:
- LCP: 1.5s (64% improvement)
- FID: 45ms (70% improvement)
- CLS: 0.02 (92% improvement)
- Conversion Rate: 2.67% (27% increase)
The best part? These improvements led to an additional $42,000 in monthly revenue.
Measuring Your Success
Set up monitoring using:
- Vercel Analytics – Real-user monitoring
- Google Search Console – SEO impact
- Chrome UX Report – Field data
- Custom Events – Business metrics
Next Steps
- Audit Your Current Performance
- Use PageSpeed Insights
- Check Chrome DevTools
- Review your Analytics
- Start with High-Impact Changes
- Focus on LCP first
- Tackle obvious CLS issues
- Optimize critical paths
- Monitor and Iterate
- Set up continuous monitoring
- A/B test improvements
- Track business metrics
Future-Proofing Your eCommerce Site
Stay Updated with Next.js
- Monitor new releases
- Implement performance-enhancing features
- Keep dependencies updated
Adapt to New Standards
- Prepare for mobile-first indexing
- Monitor new Web Vitals metrics
- Implement progressive enhancement
Need Help?
Whether you’re migrating an existing store or building a new one, Bazaar comes with:
- Built-in performance optimizations for Core Web Vitals
- Pre-configured Server Components
- Optimized image handling
- Enterprise-grade security
- Scalable architecture
Ready to transform your online store’s performance?
Get Started with Bazaar eCommerce Solution
Conclusion
Next.js provides powerful tools and features that make it easier to achieve excellent Core Web Vitals scores for your eCommerce site. By following the implementation guidelines and best practices outlined above, you can create a fast, responsive, and stable shopping experience that converts better and ranks higher in search results.
Remember that optimization is an ongoing process. Regularly monitor your metrics, stay updated with Next.js features, and continuously iterate on your implementation to maintain peak performance.